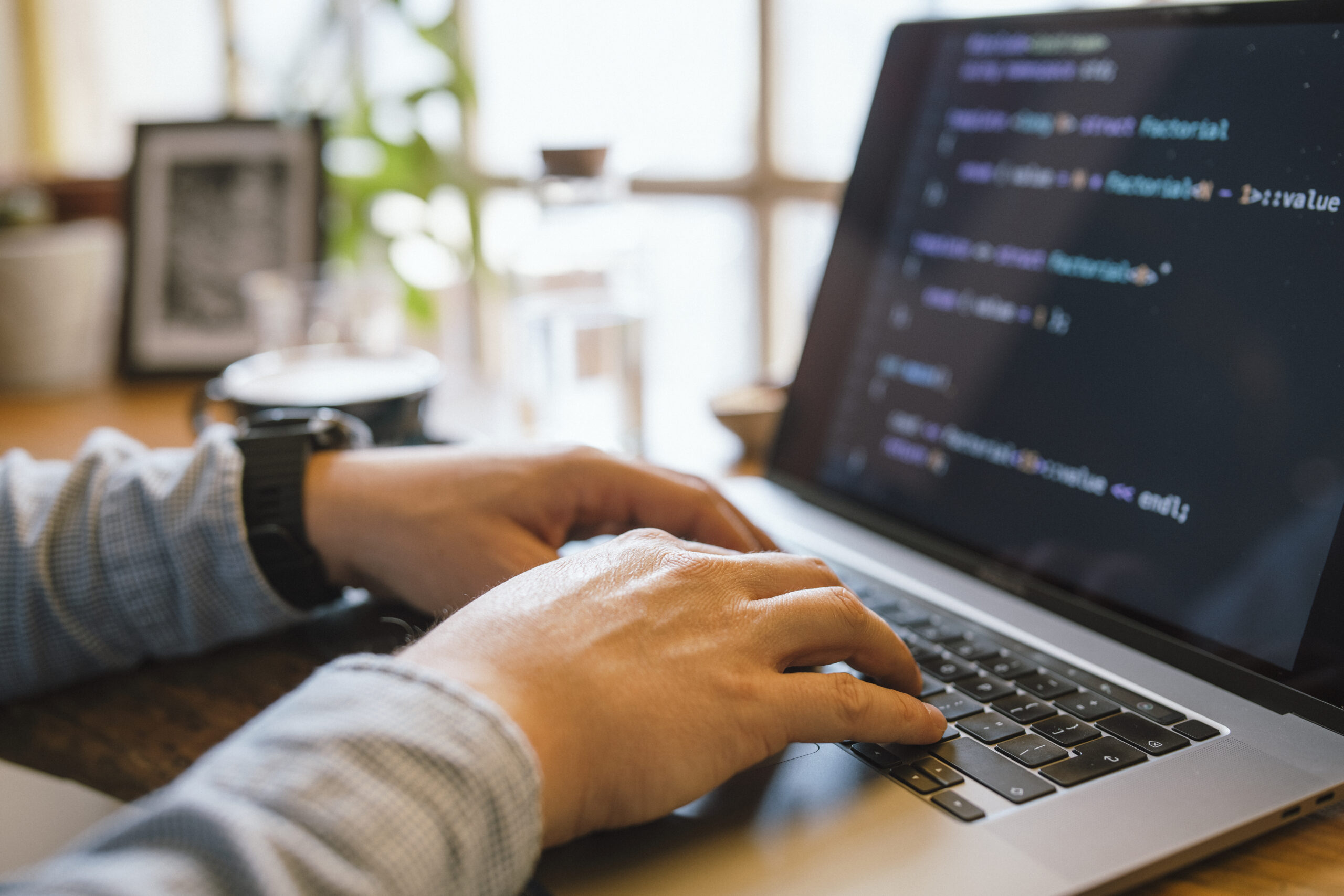
Debugging is Just about the most necessary — however usually neglected — competencies within a developer’s toolkit. It isn't really nearly correcting broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Believe methodically to solve issues effectively. No matter whether you're a novice or even a seasoned developer, sharpening your debugging techniques can help save several hours of annoyance and considerably transform your productiveness. Here are several procedures that will help builders amount up their debugging video game by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest techniques developers can elevate their debugging competencies is by mastering the tools they use every day. Though producing code is one particular Portion of development, understanding how to connect with it properly in the course of execution is Similarly significant. Modern day growth environments come Geared up with effective debugging capabilities — but lots of builders only scratch the floor of what these resources can perform.
Get, for example, an Built-in Improvement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, as well as modify code to the fly. When employed correctly, they Enable you to observe just how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer instruments, such as Chrome DevTools, are indispensable for front-close builders. They permit you to inspect the DOM, observe network requests, look at real-time functionality metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch frustrating UI challenges into manageable responsibilities.
For backend or process-level builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Manage about managing procedures and memory management. Finding out these resources may have a steeper Understanding curve but pays off when debugging general performance difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Variation control techniques like Git to understand code background, come across the precise instant bugs were introduced, and isolate problematic alterations.
In the end, mastering your equipment suggests likely beyond default settings and shortcuts — it’s about creating an intimate knowledge of your improvement setting to make sure that when challenges crop up, you’re not misplaced in the dead of night. The greater you know your resources, the more time you are able to devote fixing the actual dilemma rather then fumbling via the process.
Reproduce the trouble
The most crucial — and often ignored — actions in efficient debugging is reproducing the issue. Just before jumping in the code or generating guesses, developers require to create a constant environment or state of affairs where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of probability, typically resulting in wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as is possible. Check with inquiries like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you may have, the less complicated it gets to be to isolate the precise situations under which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your neighborhood ecosystem. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking program states. If the issue seems intermittently, consider composing automatic tests that replicate the edge conditions or point out transitions involved. These exams not only aid expose the condition but additionally protect against regressions in the future.
In some cases, the issue could possibly be environment-distinct — it'd occur only on specified working programs, browsers, or less than specific configurations. Making use of instruments like Digital machines, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for tolerance, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're currently halfway to repairing it. Using a reproducible situation, You need to use your debugging equipment additional proficiently, exam opportunity fixes properly, and communicate a lot more Plainly with all your workforce or buyers. It turns an summary criticism right into a concrete challenge — and that’s exactly where developers thrive.
Read and Comprehend the Error Messages
Error messages are sometimes the most worthy clues a developer has when something goes Improper. As an alternative to observing them as discouraging interruptions, builders must understand to deal with mistake messages as direct communications in the method. They often show you precisely what happened, where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the information very carefully and in comprehensive. Quite a few developers, especially when underneath time strain, glance at the very first line and immediately start out producing assumptions. But deeper from the error stack or logs may perhaps lie the true root bring about. Don’t just copy and paste mistake messages into serps — go through and understand them initially.
Break the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line number? What module or purpose triggered it? These inquiries can manual your investigation and place you toward the accountable code.
It’s also practical to be familiar with the terminology in the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can considerably accelerate your debugging system.
Some mistakes are imprecise or generic, and in Individuals conditions, it’s essential to look at the context by which the mistake happened. Examine similar log entries, input values, and recent improvements within the codebase.
Don’t ignore compiler or linter warnings either. These typically precede larger sized problems and provide hints about probable bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, assisting you pinpoint difficulties a lot quicker, decrease debugging time, and become a far more successful and confident developer.
Use Logging Wisely
Logging is Just about the most strong instruments inside of a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an software behaves, aiding you realize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique commences with knowing what to log and at what level. Frequent logging degrees include DEBUG, INFO, WARN, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic details for the duration of growth, Data for basic activities (like productive begin-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual complications, and Lethal if the process can’t continue on.
Keep away from flooding your logs with extreme or irrelevant information. Too much logging can obscure vital messages and slow down your program. Focus on important situations, condition modifications, enter/output values, and demanding decision details within your code.
Structure your log messages Evidently and persistently. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you monitor how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially worthwhile in creation environments exactly where stepping through code isn’t probable.
Furthermore, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. That has a effectively-thought-out logging tactic, it is possible to reduce the time it will require to spot difficulties, achieve deeper visibility into your apps, and improve the Over-all maintainability and reliability of your respective code.
Feel Similar to a Detective
Debugging is not only a complex process—it is a form of investigation. To efficiently discover and take care of bugs, developers need to technique the procedure like a detective fixing a thriller. This mindset assists break down intricate challenges into workable elements and abide by clues logically to uncover the foundation bring about.
Get started by accumulating proof. Think about the signs and symptoms of the trouble: error messages, incorrect output, or functionality difficulties. Identical to a detective surveys a criminal offense scene, acquire just as much appropriate data as you may devoid of leaping to conclusions. Use logs, take a look at scenarios, and person reports to piece together a transparent photograph of what’s going on.
Upcoming, kind hypotheses. Question by yourself: What could possibly be leading to this habits? Have any improvements not long ago been designed into the codebase? Has this challenge happened in advance of underneath related conditions? The target will be to slim down opportunities and discover likely culprits.
Then, take a look at your theories systematically. Try and recreate the situation in a very managed environment. In case you suspect a certain perform or element, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, request your code questions and Enable the outcome direct you closer to the reality.
Fork out close notice to modest particulars. Bugs normally conceal in the minimum predicted locations—similar to a missing semicolon, an off-by-just one error, or simply a race problem. Be complete and client, resisting the urge to patch the issue devoid of thoroughly knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential issues and support others recognize your reasoning.
By wondering like a detective, builders can sharpen their analytical expertise, tactic problems methodically, and turn into more practical at uncovering hidden concerns in elaborate systems.
Compose Assessments
Crafting assessments is among the most effective methods to increase your debugging competencies and overall advancement effectiveness. Assessments not simply assistance catch bugs early but in addition function a security Web that offers you assurance when creating adjustments in your codebase. A properly-examined software is easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These modest, isolated assessments can speedily expose no matter whether a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where to search, substantially decreasing the time spent debugging. Device assessments are Specifically beneficial for catching regression bugs—problems that reappear after more info Beforehand staying mounted.
Following, integrate integration tests and close-to-conclude exams into your workflow. These help make sure several areas of your application get the job done jointly effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with multiple parts or providers interacting. If something breaks, your assessments can tell you which Element of the pipeline failed and beneath what conditions.
Composing tests also forces you to definitely think critically regarding your code. To test a attribute correctly, you would like to comprehend its inputs, envisioned outputs, and edge situations. This degree of being familiar with By natural means potential customers to higher code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the check fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return in the future.
In a nutshell, writing exams turns debugging from a discouraging guessing game right into a structured and predictable method—serving to you catch a lot more bugs, speedier and much more reliably.
Get Breaks
When debugging a difficult situation, it’s quick to be immersed in the issue—watching your display screen for hrs, hoping Alternative following Remedy. But The most underrated debugging instruments is solely stepping absent. Having breaks assists you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you are also near to the code for also extended, cognitive tiredness sets in. You could start out overlooking evident glitches or misreading code you wrote just several hours previously. In this particular condition, your brain becomes less efficient at trouble-resolving. A brief stroll, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a challenge after they've taken time to disconnect, permitting their subconscious work during the qualifications.
Breaks also aid stop burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed Strength along with a clearer mentality. You would possibly abruptly notice a lacking semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
In case you’re trapped, a superb rule of thumb will be to set a timer—debug actively for forty five–sixty minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might feel counterintuitive, Specially under restricted deadlines, however it essentially leads to a lot quicker and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak spot—it’s a smart method. It presents your Mind Area to breathe, enhances your standpoint, and aids you stay away from the tunnel eyesight That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is much more than simply A short lived setback—It is a chance to mature as being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you go to the trouble to replicate and analyze what went Improper.
Start off by inquiring on your own a handful of key questions when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit tests, code reviews, or logging? The answers often expose blind places in the workflow or understanding and assist you to Develop stronger coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—which you could proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be Particularly impressive. No matter if it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same situation boosts group performance and cultivates a more powerful Studying society.
A lot more importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who publish best code, but those who repeatedly learn from their problems.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Improving your debugging expertise usually takes time, practice, and persistence — though the payoff is huge. It helps make you a far more economical, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Whatever you do.